How to Generate Unique IDs in JavaScript
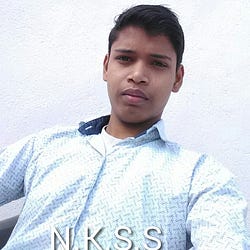
Nitish Kumar Singh
Mar 15, 2024In the modern world, where data is the most important aspect of a profitable business in the tech field, managing data efficiently and processing it in the right direction is crucial. When we have to manage, organize, and process a large amount of data records, unique identifiers (or IDs) become a necessity.
Unique identifiers serve as fundamental building blocks across various applications, facilitating seamless data management, efficient communication, and robust system architecture. They play a pivotal role in ensuring data integrity, enabling us to accurately identify and reference specific records within databases, API responses, messaging systems, and more.
Unique identifiers are crucial in many parts of modern software engineering, such as tracking user interactions on a website, managing inventory in an e-commerce platform, or handling distributed transactions in a microservices architecture.
So, hello developers! In this blog post, we are going to explore some methods of generating unique identifiers or IDs in JavaScript.
Before starting, we want to clarify one thing: generating unique IDs that are never repeated or matched exactly according to our requirements and desires is next to impossible. For example, if we aim to generate IDs using characters A-Z, a-z, 0-9, and perhaps even some additional characters, and we also desire that they never match, this goal is not achievable.
Because achieving absolute uniqueness in identifiers is constrained by the limitations of computational systems and the probabilistic nature of random generation. While we can employ various techniques to significantly reduce the likelihood of collisions, such as using large character sets, cryptographic hashing, or combining multiple factors in ID generation.
So, following are some methods of generating unique IDs with their own limitations and benefits.
Using Date.now()
This is the easiest and most accurate way of generating unique IDs in JavaScript. We just need to call the static method of the built-in JavaScript class, Date, and we get a 13-digit number. It is almost unique because it is time-dependent.
const id = Date.now();
We can also easily convert it to a string and hexadecimal string to use it in string type.
const stringId = Date.now().toString();
const hexaString = Date.now().toString(16);
console.log("stringId = "+stringId);
console.log("hexaString = "+hexaString);
stringId = 1710501412147
hexaString = 18e41d43d33
Because of base 16 in hexadecimal, the length of hexaString is 11, but the length of stringId is 13. This method may fail when a large number of IDs are created with different computing systems and can be reduced by generating IDs with a single system. IDs generated by this method only include 0-9 and a-z.
Using Math.random()
This is another method of unique ID generation. First, we generate a random decimal number between 0 to 1, convert it to a hexadecimal string, and then remove the first two characters ("0.") from the string.
const random = Math.random(); // id = 0.1930028261207366
const s = random.toString(16); // s = '0.3168a21a39612'
const id = s.slice(2); // id = '3168a21a39612'
// In one line
const id = Math.random().toString(16).slice(2);
In this method, there is a higher chance to match two generated IDs, and this method also includes only 0-9 and a-z.
Using an ID Generator Function
This is a more flexible method of ID generation. In this method, we control the length of the ID and characters included in it, and there is less chance of repeating because each character of the ID is selected randomly from 62 characters.
const generateUniqueId = (length)=> {
const characters = 'abcdefghijklmnopqrstuvwxyzABCDEFGHIJKLMNOPQRSTUVWXYZ0123456789';
let id = '';
for (let i = 0; i < length; i++) {
const randomIndex = Math.floor(Math.random() * characters.length);
id += characters[randomIndex];
}
return id;
}
We can also reduce the chance of repeating by including time-dependent characters in it and making it more unique.
const generateUniqueId = (length)=> {
const characters = 'abcdefghijklmnopqrstuvwxyzABCDEFGHIJKLMNOPQRSTUVWXYZ0123456789';
let id = '';
for (let i = 0; i < length; i++) {
const randomIndex = Math.floor(Math.random() * characters.length);
id += characters[randomIndex];
}
return Date.now().toString(16) + id;
}
These are some methods to generate IDs in JavaScript, and I am researching to create a function that generates IDs based on time with all 62 characters mentioned above, and I will list it here after generation.
I hope you find this post helpful and informative. For any suggestions, ideas, and questions, feel free to comment below. Until next time, happy coding!