Four Ways to Remove All Children from an HTML Element in JavaScript
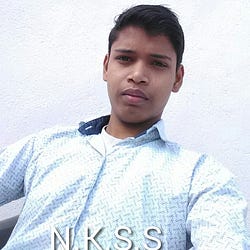
Nitish Kumar Singh
Dec 7, 2023Hello, developers! In this blog post, we will explore 4 methods for removing all children, including elements, texts, and comments, from an element in JavaScript.
Using innerHTML
We can remove all children, including text nodes, from an element using the innerHTML
property of the Element. This is a good way to remove all children because it removes them all at once. So the browser does not need to repaint repeatedly on every child removal.
var element = document.getElementById("parent");
element.innerHTML = "";
Using textContent
Similar to innerHTML
, we can also remove all children from an element at once using the textContent
property.
var element = document.getElementById("parent");
element.textContent = "";
Using firstChild and while loop
We can remove all children from an element using the firstChild
property of the Element and a while loop. We run a while
loop with the condition element.firstChild
and remove nodes using the remove()
method on the child or removeChild()
on the parent element. This is not an efficient way to remove all children because in this way, removal is done one by one. However, it is the right way if you want to keep some children by checking some conditions.
var element = document.getElementById("parent");
while (element.firstChild) {
element.removeChild(element.firstChild);
// OR
element.firstChild.remove();
}
Make sure to use element.firstChild, not element.firstElementChild
because it only removes element nodes, not text nodes.
Using replaceChildren
With replaceChildren
, we can remove all children at once with the advantage of keeping some children if needed. This method not only removes children from one element but also allows us to move children from one element to another.
var element = document.getElementById("parent");
// To remove all childs call replaceChildren method with no parameters
element.replaceChildren();
// To remove all but not first and last childs
element.replaceChildren(element.firstChild,element.lastChild);
The first two ways are best if you want to remove all children at once, and the last two ways are best to remove all children while filtering some children.
I hope you understand all the ways of removing all children from an element and enjoy reading this blog post. Happy Coding!