20 DOM Methods Every Developer Should Know
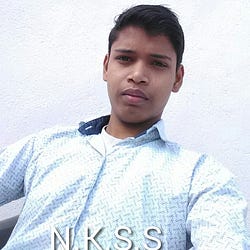
Nitish Kumar Singh
Dec 6, 2023Hello developers! In this blog post, we will explore 20 fundamental DOM methods that every developer should be familiar with.
The Document Object Model (DOM) is a crucial aspect of web development, serving as a programming interface for web documents. As a developer, mastering DOM methods is essential for manipulating and interacting with the structure, style, and content of web pages dynamically.
The following methods are used to get an element or a set of elements based on their type, properties, property values, or their position on the screen relative to the viewport.
getElementById
The getElementById
is a basic method of DOM manipulation. It allows us to select and access a specific HTML element by its unique identifier (id). This method is efficient and widely used for targeted interactions with elements on a page.
let element = document.getElementById('myElement');
getElementsByClassName
The getElementsByClassName
method, we use to retrieve a collection of elements based on their class name. It's useful for applying changes to multiple elements that share a common class.
let elements = document.getElementsByClassName('myClass');
getElementsByTagName
With getElementsByTagName
, we can select elements based on their tag name. This method is handy when dealing with a group of elements of the same type.
let paragraphs = document.getElementsByTagName('p');
querySelector
The querySelector
method allows us to select the first element that matches a specified CSS selector. It provides a powerful way to access elements with more complex criteria.
let element = document.querySelector('.myClass');
querySelectorAll
Similar to querySelector
, querySelectorAll
returns a NodeList containing all elements that match a specified CSS selector. This is useful for handling multiple elements at once.
let elements = document.querySelectorAll('.myClass');
elementFromPoint
The elementFromPoint
method allows us to retrieve the topmost element at a specified set of coordinates on the page. This is beneficial for scenarios where precise interactions based on user mouse or touch events are required.
let element = document.elementFromPoint(x, y);
I have written a blog post on an interesting use case of this method, explaining how to "Retrieve Element Intended for Click in JS, But Click Event Canceled by Java Code in WebView."
The following methods are used to create a new node, append (add) it to a parent element either at the end, before, or after a specific node. These methods also used to remove an element and the replacement of children within an element, either from one element to another or by emptying it.
createElement
The createElement
method is essential for dynamically creating HTML elements in JavaScript. We can then manipulate and append these elements to the DOM as needed. This method is widely used when creating Single Page Applications.
let newElement = document.createElement('div');
appendChild
This method we used to insert a new child element into an existing parent element at the end. It's commonly used to dynamically add content to a webpage.
parentElement.appendChild(newElement);
after
The after
method, part of the ParentNode interface, inserts a set of Node objects or DOMString objects immediately after the specified child.
element.after(newChild1, newChild2, ...);
before
Similar to after
, the before
method inserts a set of Node objects or DOMString objects immediately before the specified child.
element.before(newChild1, newChild2, ...);
replaceChildren
The replaceChildren
method simplifies the process of replacing the children of an element with a new set of nodes. This can be particularly useful when updating the content of a container element dynamically and removing all children.
parentElement.replaceChildren(newChild1, newChild2, ...);
You can explore more about how to remove all children of an element, replace elements with new ones, and transfer children from one element to another.
remove
The remove
method removes the specified node from the DOM. This is a cleaner alternative to setting parentNode.
removeChild(node)
.
element.remove();
The following methods are used to dynamically manipulate the attributes and styles of an element, enabling dynamic and responsive adjustments in a web application.
setAttribute
The setAttribute
method allows us to set or change the value of an attribute on a specific element, providing flexibility in modifying HTML elements. And removeAttribute
method use to completely remove attribute of an element.
element.setAttribute('src', 'new-image.jpg');
classList
The classList
property provides methods like add, remove, and toggle to manipulate the classes of an element easily. This is particularly useful for dynamic styling.
// add a css class
element.classList.add('newClass');
// remove a css class
element.classList.add('newClass');
The following methods are used to dynamically scroll a web page or a scrolling container, facilitating smooth and controlled navigation or presentation of content.
scrollIntoView
The scrollIntoView
method is a convenient way to smoothly scroll the specified element into the visible area of the browser window. This is especially useful when dealing with long pages or dynamically loaded content.
element.scrollIntoView({ behavior: 'smooth' });
scrollBy
The scrollBy
method allows us to scroll a specified amount from the current scroll position. This is useful for implementing smooth scrolling effects or responding to user interactions.
window.scrollBy({
top: 100,
left: 0,
behavior: 'smooth',
});
The following two methods are used to listen for user interactions, enabling actions to be taken based on these interactions or preventing them when necessary.
addEventListener
With addEventListener
, we can attach event handlers to elements, so we can listen for user interactions and take actions accordingly.
element.addEventListener('click', function() {
// Handle click event
});
preventDefault
While not a method of the DOM itself, the preventDefault
method is commonly used in event handlers to prevent the default action associated with an event, such as preventing a form from submitting or a link from navigating. This can be considered the essence or core of Single Page Applications.
element.addEventListener("click",(event)=>{
event.preventDefault();
});
I have explained how this method plays a crucial role in the development of Single Page Applications. You can learn more about SPAs by reading the blog posts: "Understanding Single Page Applications in Pure JavaScript" and "Creating Single Page Applications in Pure JavaScript." Additionally, you can visit the Text-Tools website, which was developed without using any library or framework.
animate
The animate
method is used for smoothly animating changes to CSS properties. It returns an Animation object that can be controlled and queried for information about the animation.
element.animate(keyframes, options);
Explore the blog post "How to Create a Toast or Snackbar using CSS and JavaScript" that explains a use case of this method to create a Toast or Snackbar.
requestFullscreen
The requestFullscreen
method allows us to make an element take up the full screen. This is particularly useful for immersive web experiences or media playback.
element.requestFullscreen();
A solid understanding of these twenty DOM methods is crucial for us to create dynamic and interactive web applications. These methods form the foundation for manipulating the DOM, enabling us to build powerful and responsive user interfaces. As you continue to explore web development, mastering these methods will empower you to create more efficient and feature-rich applications.
I hope this blog provide you with valuable insights into essential DOM methods, improving your web development journey. May these tools become the building blocks for crafting seamless, interactive, and user-friendly applications. Happy coding!