How to create Toast or Snackbar using CSS and JavaScript
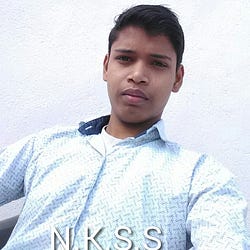
Nitish Kumar Singh
Nov 10, 2023 . 4 min readHello Developers! In this blog post, we will create a Toast or Snackbar using CSS and JavaScript to show a short-duration, non-interactive message to the user upon an action. We will design the Toast to be versatile, serving as an informational, warning, error, or success message by utilizing different colors for each message type. Additionally, we'll explore the customization of the Toast's position on the screen.
What is Toast or Snackbar?
A Toast, Snackbar, or similar component is a non-interactive UI element used to display a message to the user for a short time span. Components like these are employed to show brief messages or notifications without requiring user interaction. They appear on the screen with an animation, delivering a concise message, and automatically dismiss after a short period without any user interaction. Typically, we use them to convey messages in the following scenarios:
- Form Submission Success/Failure: After a user submits a form, we can display a Snackbar to confirm success or inform about an error.
- User Authentication: To confirm successful login or notify about authentication errors.
- Data Updates: When data is successfully updated or if there's an issue during the update process.
- Alerts or Warnings: For non-critical warnings or alerts that don't require a full page refresh.
- Network Actions: When performing actions that require an internet connection, like syncing data or sending a message.
- Product Added to Cart: In e-commerce websites, showing a Snackbar when a user adds a product to their cart.
Above are common use cases where we use components like this, but they can be employed in many more situations. It completely depends on the use case where and how to use them.
So, without any more theoretical introduction, let's start building. Add the following CSS to your CSS file:
.toast {
position: fixed;
margin: 10px;
border-radius: 5px;
color: white;
padding: 10px;
font-size: 15px;
letter-spacing: 0.4px;
font-family: sans-serif;
}
Customize above CSS as your requirements, like margin, font styling and boder-radius but keep position fixed because you change it then you need to change over all JavaScript code.
Add following JavaScript to a JS file:
const types = { info: "dodgerblue", warn: "darkorange", error: "red",success:"forestgreen" };
export const showToast = (o)=> {
if (!o) o = {message:"Empty message"}
const { message, time, from, type } = o;
const m = document.createElement("div"); m.classList.add("toast");
const keyframes = [];
let s = "";
switch (from) {
case "tl": case "tr":
keyframes.push({ transform: "translateY(-100%)", top: 0 });
s = from === "tl" ? "let:0;top:0;" : "right:0;top:0;"; break;
case "bc":
keyframes.push({ transform: "translateY(100%)", bottom: 0 });
s = "right:10px;bottom:0;left:10px;width:fit-content;margin:30px auto;"; break;
case "bl": case "br": default:
keyframes.push({ transform: "translateY(100%)", bottom: 0 });
s = from === "br" ? "right:0;bottom:0;" : "left:0;bottom:0;"; break;
}
s = s +"background:" + types[type || "info"] + ";box-shadow: 0 0 10px 2px " + types[type || "info"] + ";";
keyframes.push({ transform: "translateY(0px)" });
m.style = s;
m.textContent = message || o;
document.body.appendChild(m);
m.animate(keyframes, { duration: 300 })
.onfinish = () => {
m.animate(keyframes, {delay: time || 1000,duration: 300,direction: "reverse"})
.onfinish = () => {
m.remove();
};
};
}
And use it, In any where in your web app using following code:
// import showToast function by replacing ... with relative path
import {showToast} from '...';
// To show an info message just pass message to showToast function
showToast("This is an info message");
The showToast
function accepts either a String or an Object as arguments. To display a simple informational message, pass a String as the message. For more customization, pass an Object with the following properties:
message
: A string to be displayed as a message or notification.time
: The duration, in milliseconds, for which the message is displayed before automatic dismissed. The default duration is 1000 milliseconds (1 second).from
: The position of the Toast on the screen. Usebl
for bottom-left corner,br
for bottom-right corner,tl
for top-left corner,tr
for top-right corner, andbc
for bottom-center. The default position is bottom-left.type
: The type of message. Useinfo
for informational,warn
for warming,error
for error andsuccess
for successful messages. The default is informational message. The colors used for message types as follows:{ info: "dodgerblue", warn: "darkorange", error: "red", success: "forestgreen" }
, you can customize it in first line of JavaScript code.
In this component, I have used slide animations for its dynamic presentation. However, feel free to customize the animation further by modifying the CSS and JavaScript code according to your preferences and requirements.
Alright, I've covered the fundamental aspects, I believe I've addressed the common and necessary points. I hope you'll find this blog post enjoyable and informative, and that it provides you with valuable insights into creating dynamic and customizable Toasts or Snackbars. Happy reading!