How to Remove All Child Elements at Once in JavaScript
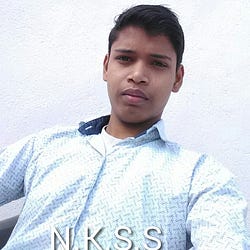
Nitish Kumar Singh
Nov 17, 2023Hello, developers! In this blog post, we will learn how to remove all child elements at once from an HTML element using JavaScript. Removing all children at once is a little problematic task on the web because the web does not have any method like removeAll() that removes all children.
It is correct that the web (DOM) does not have any method specifically for this purpose, but it has a more advanced method, which is replaceChildren() of the HTML Element. As the name suggests, replaceChildren()
is used to replace all children with new children passed as parameters. This method takes nodes or strings as parameters, like replaceChildren(node1, node2, ...., nodeN)
.
We use this method for many purposes, and here are a few examples:
Remove All Child at Once
Just call this method with zero params on the element from which you want to remove all children. The code below is an example:
const yourTargetElement = document.getElementById("yourId");
yourTargetElement.replaceChildren();
Remove Only a Few Children
We can use it to remove only some children or remove all except for some children. Below is the code for removing all children except the first and last ones:
const target = document.getElementById("yourId");
target.replaceChildren(target.firstElementChild,target.lastElementChild);
Replace Children from One Element to Another
We can also use it to move children from one element to another. The following code is an example of this:
const parent1 = document.getElementById("oneElement");
const parent2 = document.getElementById("otherElement");
// let's assume parent1 have five childs and want to send last three childs to parent2
let targetElements = [...parent1.children].splice(2,3);
parent2.replaceChildren(...targetElements);
Before I learned about this method, I tried many ways to remove all children except the first and last (if it is a button element) and gained some insights into the DOM. Here, I will mention one attempt.
Initially, I attempted to achieve this using a for loop, and the following is the trial code:
var resContainer = document.querySelector("main").firstElementChild.firstElementChild.firstElementChild.firstElementChild.firstElementChild;
let childs = resContainer;
for(let i=0;i<childs.length;i++){
if(i>0){
const element = childs.item(i);
if(element.nodeName === "DIV"){
element.remove();
}
}
}
After running this code multiple times, I noticed that only half of the children were being removed with each execution and wondered why it happened. After adding log statements, I gained an understanding of the reason behind this behavior. You can also comprehend it by referring to the logging screenshot below.
So, I realized that DOM methods return objects that are internally stored. Consequently, removing nodes also removes items from the collection returned by the children property. As depicted in the image, the length of the collection decreases as nodes are removed one by one.
I hope you now understand how to remove all children at once in JavaScript and have enjoyed reading this blog post.