How to List All Videos in Android App Development using Java
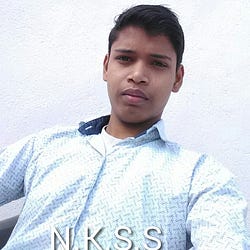
Nitish Kumar Singh
Nov 25, 2023Hello Developers! In this blog post, we will learn how to list or extract all video files with information from Internal (Phone) and External (SD Card) storages in Android app development using Java. For example, if we are developing a Video Player app, we need to show all videos.
To get all video files, we first obtain absolute paths of all storages available on the device. I explained how to get these paths in my previous blog post. So, we use extracted root paths, not hardcoded paths. After that, we use listFiles
and some methods of the File API (class) and a for loop to recursively loop over root paths and filter video files by extensions.
We use the following steps to complete our work:
- Get Root Paths:
Let's obtain root paths as an object of
ArrayList<String>
with the object's namerootPaths
. I explained how to get it in my previous blog post. - Video File Extensions:
To list videos, we need a list of video extensions that we want to include. Let's have an object named extensions of
ArrayList<String>
that contains items like.mp4
,.mkv
,.webm
, and more. We need to add all extensions of video files to this list. - Using File API:
The rest of the work is done using the
File
class, its methods, and a for loop. You will automatically understand the rest by seeing the codes provided in this blog post.
We create two classes: one is FilesExtractor
for doing video scanning, and the second is VideoFile
for storing video information. Here, I put all scanning codes in a class, but you can keep it in an Activity or however you want to use it.
The following code is of the VideoFile
class where title
, path
, size
, and lastModified
fields store video title, its absolute path, size in bytes, and last modified date in milliseconds, respectively:
public class VideoFile {
public String title,path;
public long size,lastModified;
public VideoFile(String title, String path, long size, long lastModified) {
this.title = title;
this.path = path;
this.size = size;
this.lastModified = lastModified;
}
}
The following code if of FilesExtractor
class:
public class FilesExtractor {
private final Context context;
private ArrayList<VideoFile> videoFiles;
private final ArrayList<String> defaultVExtensions = new ArrayList<>();
public FilesExtractor(Context context) {
this.context = context;
defaultVExtensions.add(".mp4");
defaultVExtensions.add(".mkv");
defaultVExtensions.add(".webm");
defaultVExtensions.add(".mpg");
defaultVExtensions.add(".3gp");
defaultVExtensions.add(".avi");
defaultVExtensions.add(".tc");
}
public void addMoreExtensions(ArrayList<String> e){
defaultVExtensions.addAll(e);
}
private ArrayList<String> getRootPaths() {
ArrayList<String> rootPaths = new ArrayList<>();
File[] rootsStorage = ContextCompat.getExternalFilesDirs(context, null);
for (File file : rootsStorage) {
String root = file.getAbsolutePath().replace("/Android/data/" + context.getPackageName() + "/files", "");
rootPaths.add(root);
}
return rootPaths;
}
public ArrayList<VideoFile> listVideos(){
videoFiles = new ArrayList<>();
ArrayList<String> rootPaths = getRootPaths();
for (String path:rootPaths){
getVideos(new File(path));
}
return videoFiles;
}
private void getVideos(File folder) {
File[] folders1 = folder.listFiles();
if (folders1!=null){
for (File file :folders1){
String name = file.getName();
if (file.isDirectory()){
getVideos(file);
}else {
int i = name.lastIndexOf(".");
String exe = i == -1 ? "" : name.substring(i);
if (defaultVExtensions.contains(exe)) videoFiles.add(new VideoFile(file.getName(),file.getAbsolutePath(),file.length(),file.lastModified()));
}
}
}
}
}
This class code is described in the following points:
- FilesExtractor: This constructor takes context as params for root paths extraction and default extensions in the list.
- addMoreExtensions: This method is used to add more video extensions.
- getRootPaths: This method lists paths of all storages, where the first path is for Phone storage and the second, if available, is for the SD Card.
- listVideos: This is the method that we call to get a list of all video files.
- getVideos: This is the main method that performs scanning of storages by looping over folder to folder. Here, we get all files and folders as
Files[]
and check if it's notnull
.Files[]
contains both folders and files, so we loop over this array. If a file is a folder, we call thegetVideos
method again. If the file is a file, we get its title, extract the extension, and check if it's a video by searching in the extension list. If yes, then we add it to our video list.
Whenever we need to list video files, we just use the following code:
FilesExtractor filesExtractor = new FilesExtractor(MainActivity.this);
// call if need to add more extensions
// filesExtractor.addMoreExtensions(moreExtensions);
ArrayList<VideoFile> videoFiles = filesExtractor.listVideos();
// now these video files we can show in RecyclerView
In this way, we can also list any type of files like audio, documents, images. Just change the extensions list as needed.
I hope this blog post has provided you with a clear understanding of how to list video files in Android app development using Java. By following the outlined steps, you can easily extract information about video files from both internal and external storage locations on the device. The code snippets provided for the FilesExtractor and VideoFile classes offer a practical implementation that can be integrated into your projects. Whether you are developing a Video Player app or dealing with various file types, this approach can be adapted by modifying the extensions list. Happy coding!