How to Get Absolute Paths of Internal and External Storages in Android Development
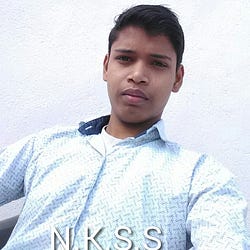
Nitish Kumar Singh
Nov 24, 2023Hello developers! In this blog post, we will learn how to get the absolute paths of Internal (Phone storage) and External (SD Card) storages in Android app development using Java.
When developing a File Manager, Video Player, Music Player, or any app where we need to list all or a specific type of files, we need to retrieve paths of Internal and External storages.
We know that the path of Internal storage is /storage/emulated/0
, and External storage is /storage/name_of_sd_card
in almost all devices. Here, name_of_sd_card
is a variable because it is not the same for all SD Cards. Using these hard-coded paths is not a good idea.
So, how do we get paths because the Android SDK does not provide any direct methods to obtain paths? We can list files with MediaStore
, but here we want to use the File API.
To get paths, we use the ContextCompat.getExternalFilesDirs
method. This method returns paths to the files directory (application-specific) on all available external storage devices. The returned paths are not exactly what we want, but we can modify them to suit our needs. See the following lines to understand what the Android Developer Documentation says about this method.
Returns absolute paths to application-specific directories on all external storage devices where the application can place persistent files it owns. These files are internal to the application, and not typically visible to the user as media.
The following code shows how we obtain our desired paths:
ArrayList<String> rootPaths = new ArrayList<>();
File[] rootsStorage = ContextCompat.getExternalFilesDirs(context, null);
for (int i = 0; i < rootsStorage.length; i++) {
String root = rootsStorage[i].getAbsolutePath().replace("/Android/data/" + context.getPackageName() + "/files", "");
rootPaths.add(root);
}
Here, the first path is for Phone Storage, and the second path, if available, is for the SD Card. The returned paths do not include USB storage. To list a specific type of files, we loop over these files and filter with extensions to get the required files.
I hope you learn how to obtain paths of Internal and External storages and enjoy reading this blog post.