How to Change Brightness of Only One Activity in Android Using Java
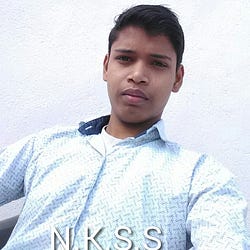
Nitish Kumar Singh
Nov 13, 2023Hello Developers! In this blog post, we will learn how to change the brightness of only one activity, not for the overall application or the entire phone brightness. For example, if the user drags on the screen, changes the progress of the seek bar, or clicks on buttons, then the brightness of the activity should change. This is a feature commonly seen in Video-Player Apps.
Note: In this post, I will demonstrate only which fields or classes we need to use for this purpose, how to use them, and provide an overview of achieving this by dragging on the screen and changing the progress of the seek bar.
To implement this feature, we need to modify the screenBrightness
field of WindowManager.LayoutParams
between zero (lowest brightness level) to one (highest brightness level) in the following steps:
- Get an object (let's call it window) of
Window
by calling thegetWindow()
method of Activity. - Obtain an object of
WindowManager.LayoutParams
(let's call it layoutParams) by calling thegetAttributes()
method of theWindow
class on the window object. - Change the
screenBrightness
field of thelayoutParams
object according to user interaction, ranging from zero (dark) to one (full bright). Setting any value less than zero changes the activity brightness to the user's preferred brightness of the screen. - Finally, set this modified
layoutParams
object on the window object by calling thesetAttributes()
method ofWindow
.
Here's the code for this:
Window window = getWindow();
WindowManager.LayoutParams layoutParams = window.getAttributes();
layoutParams.screenBrightness = value; // where value is a float from 0 to 1;
window.setAttributes(layoutParams);
The WindowManager.LayoutParams
class has many more fields to experiment with. You can explore more about this class here, and below are the docs about the screenBrightness
field from the Android Developer Documentation.
This can be used to override the user's preferred brightness of the screen. A value of less than 0, the default, means to use the preferred screen brightness. 0 to 1 adjusts the brightness from dark to full bright.
Overview of Implementing This with Different Methods:
- Using Seekbar: Implementing this with a SeekBar is easy. Just add
SeekBar.OnSeekBarChangeListener
to theSeekBar
and change thescreenBrightness
field in theonProgressChanged
method of this listener. - Using Drag on Screen: Implementing this with dragging on the screen is a bit more challenging. Set
View.OnTouchListener
on a view that covers almost the entire screen. In theonTouch
method of this listener, get touch points of the pointer (finger) on every touch event, store it for the next event, and process it to make it fall in the range of 0 to 1 for setting as the value of thescreenBrightness
field. Note that it can be challenging because touch events are fired rapidly, and the difference between two consecutive touch points is almost greater than one.
I hope you enjoyed this blog post and learned how to change the brightness of an activity in Android app development. Get an idea of doing it by using a seekbar or dragging on the screen.