How to Convert Milliseconds Time into Formatted String (HH:MM:SS) in Java
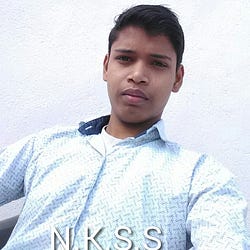
Nitish Kumar Singh
Nov 12, 2023 . 3 min readHello Developers! in this blog post, we will learn how to convert milliseconds time into formatted String in Java. For example 6548000 milliseconds into 1:49:08, so we can show it as duration to user.
When do we need to do this?
For example when we developing a Video or Audio Player or any application where need to show time or duration of video or audio then we need to do this because when we extract duration of video or audio files then generally it extracted in milliseconds. So we need to convert it in String format like in HH:MM:SS format or in x Hours y Minutes z Seconds and show it to users.
So let's start coding, To do this we use two Java operators: division(/) and modulus(%) to calculate Hours, Minutes and Seconds, and StringBuilder and Formatter to formats it in HH:MM:SS format.
So below is a Java method that take time in milliseconds and return time in HH:MM:SS formatted String.
private String getTimeInHhMmSsString (int timeMs) {
int totalSeconds = timeMs / 1000;
int seconds = totalSeconds % 60;
int minutes = (totalSeconds / 60) % 60;
int hours = totalSeconds / 3600;
StringBuilder mFormatBuilder = new StringBuilder();
Formatter mFormatter = new Formatter(mFormatBuilder, Locale.getDefault());
mFormatBuilder.setLength(0);
if (hours > 0) {
return mFormatter.format("%d:%02d:%02d", hours, minutes, seconds).toString();
} else {
return mFormatter.format("%02d:%02d", minutes, seconds).toString();
}
}
Explanation Points:-
- modulus(%) operator: This operator gives remainder when one integer divided by other. So
totalSeconds % 60
gives seconds as remainder because hours and minutes are multiple of 60,(totalSeconds / 60) % 60
gives minutes as remainder (because let totalSeconds = 120 then 120/60 = 2 and 2 % 60 = 2) andtotalSeconds / 3600
gives hours becausehours
is an int variable. - StringBuilder: This class use to build string by appending multiple strings by it's
append()
method. - Formatter: This class use to formats string by using a format specifier (string), arguments according to specifier and a Locale. In the above code specifier are
"%d:%02d:%02d"
and"%d:%02d"
and arguments are hours, minutes, seconds. Here%d
means formats the integer (d mean decimal or integer) and%02d
means - format the integer with 2 digits and padding is zero like if argument is 4 then formatted result is 04.
And below is a Java method that take time in milliseconds and return time in hours, minutes, seconds formatted String.
private String getTimeInHoursMinSec(int timeMs) {
int totalSeconds = timeMs / 1000;
int seconds = totalSeconds % 60;
int minutes = (totalSeconds / 60) % 60;
int hours = totalSeconds / 3600;
if (hours > 0) {
return hours>1?hours+" hours":" hour" + minutes>1?minutes+" minutes":" minute" + seconds>1?seconds+" seconds":" second";
} else {
return minutes>1?minutes+" minutes":" minute" + seconds>1?seconds+" seconds":" second";
}
}
In the above code, I use Java Ternary Operator(?). Syntax of ternary operator is condition ? value on true : value on false
, like in above code if hours greater than 1 then " hours" else " hour" used as value in string concatenation.
Alright, I hope you enjoyed this blog post and learned how to convert milliseconds time into formatted string.