How to Convert Binary Number to Decimal Number in Java
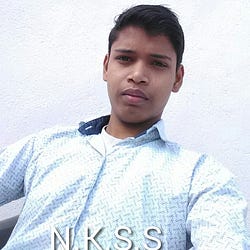
Nitish Kumar Singh
Jan 15, 2025Converting a binary number to a decimal number is a basic operation in coding that helps us understand binary numbers and their connection to decimal numbers. In this post, we will learn what a binary number is, the steps to convert it to decimal, and write a Java method to perform this task.
What is a Binary Number?
A binary number is a number expressed in the base-2 numeral system, which uses only two digits: 0 and 1. Each digit in a binary number is referred to as a bit, and the value contribution of each bit in decimal conversion depends on its position. This value is the product of the digit itself and a power of 2, with powers starting from 0 at the right side. For example, the binary number 1011 can be expressed in decimal as:
1 × 2³ + 0 × 2² + 1 × 2¹ + 1 × 2⁰ = 8 + 0 + 2 + 1 = 11
For example, the extended form of the decimal number 78957 is as below:
7 × 10⁴ + 8 × 10³ + 9 × 10² + 5 × 10¹ + 7 × 10⁰
In a decimal number, each digit has a place value of the product of the digit itself and a power of 10, with powers increasing from 0 at the right side. In the decimal system, the base is 10, but in the binary system, it is 2.
Therefore, the number system with base 10 (decimal) is called the decimal or denary number system, and the number system with base 2 (binary) is called the binary number system.
To convert a binary number to a decimal number, we need to take each digit from that number starting from the right side, multiply it by a power of 2 (starting from 0), repeat this for all digits, and add all values to get the final decimal number.
Here question is that how to get last digit from a number and remove that digit from it? so we can perform above operations for all digits.
How to Get the Last Digit From a Number
To get the last digit of a number, we divide it by 10, and the remainder is our last digit. For example, when we divide 89 by 10, the remainder is 9, which is the last digit of 89. We can get the last digit in Java using the modulus operator as shown below:
int number = 89;
int lastDigit = number % 10;
How to Remove the Last Digit From a Number
We can remove the last digit from a number by dividing it by 10. For example, when we divide 89 by 10, the result is 8, which is the number after removing 9 from 89.
int number = 89;
int numberAfterRemovingLastDigit = number / 10;
Putting It All Together
To convert a binary number to decimal, we need to:
- Get the last digit of the binary number.
- Multiply it by a power of 2.
- Add it to a variable storing the decimal value.
- Remove the last digit from the binary number.
We repeat these steps for all digits. To achieve this, we run a while
loop until the given binary number becomes 0. By dividing the binary number by 10 in each loop iteration, it eventually becomes 0 after n iterations, where n is the number of digits in the binary number.
Java Method: binaryToDecimal
Finally, all our above logical discussions result in a Java method binaryToDecimal
, as shown in the code below.
public class JavaBasics {
public static void main(String[] aStrings){
System.out.println(binaryToDecimal(0));
System.out.println(binaryToDecimal(01));
System.out.println(binaryToDecimal(10));
System.out.println(binaryToDecimal(11));
System.out.println(binaryToDecimal(100));
System.out.println(binaryToDecimal(101));
System.out.println(binaryToDecimal(110));
System.out.println(binaryToDecimal(111));
System.out.println(binaryToDecimal(1000));
}
public static int binaryToDecimal(long binary){
if(binary < 0 || !(""+binary).matches("[01]+")) throw new IllegalArgumentException("Invalid binary number");
int decimal = 0, power = 0;
while (binary>0) {
int lastDigit = (int) binary % 10;
decimal += lastDigit * (int) Math.pow(2, power);
binary = binary / 10;
power++;
}
return decimal;
}
}
In the above code, the first line of the binaryToDecimal
method checks if the given binary number is valid or not and processes it accordingly.
This is how we can write a method in Java to convert a binary number to a decimal number. I hope you found this post helpful, informative, and enjoyable to read. Happy Coding!