How to Save Content of a Web Page Element as PDF using JavaScript
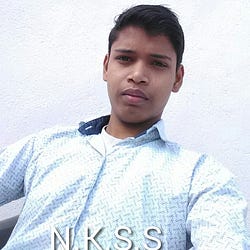
Nitish Kumar Singh
Feb 16, 2024Hello developers! In this blog post, I will show you a solution to a question that I have recently faced: how can we save or create a PDF file from the content of a web page element using JavaScript?
Recently, I updated my resume on resume.io (a perfect resume writing tool or website). When I tried to save or get the resume in PDF format, resume.io redirected me to a subscription page to pay for saving the resume as PDF.
However, I wanted to get my resume as a PDF without paying. So, I searched Google for how to save the content of an element as a PDF or how to make a PDF in JavaScript, and I found out about PDFKit.
PDFKit is a JavaScript library to create PDF documents with text, images, shapes, and more. Using it, we can create complex PDF documents with annotations. It can be used in both Node and browser environments.
So, I decided to use it to get my resume as a PDF. When I tried to use it in the browser with the provided instructions in the documentation, I encountered an error and ended up doing my work in the Node environment.
Now, I will describe the process that I used to complete my work, but you can customize it according to your needs if you want to perform a similar task. I want to mention one more thing: this solution is good for a coder who is curious to learn something new because it helps them solve their problem and learn something in the process.
Before starting, make sure you have Node.js and VS Code installed. Open an empty or Node project folder in VS Code, then install PDFKit in your empty Node.js project or create a new one, and install PDFKit by running the following command:
// If you need to create new js project
npm init --y
// Install PDFKit with below command
npm install pdfkit
Create a new JS file like index.js
and create an instance of PDFDocument
for creating a PDF file using the code below in the index.js
file:
const PDFDocument = require('pdfkit');
const doc = new PDFDocument();
This creates a new PDF document, and PDFKit automatically adds a page. We can provide the page size and margin for all pages in the document when creating the doc object. For example, I have used the code below to get the right PDF file:
const doc = new PDFDocument({size:[900,1272.5],margin:0});
Now we have a one-blank-page PDF document. We can add our content to the PDF's page with full customization using PDFKit's provided functions. You can find a full list of functions in their documentation and use them as needed. I have used the code below to get my resume PDF:
doc.image("resume-image.jpg",0,0,{width:900});
l.map((link)=>{
doc.link(link.x, link.y, link.w, link.h, link.url);
});
Where the image()
function takes the path of the image, x and y coordinates, and an option object with properties as needed. Resume.io shows the resume in just one image with all content except links, so I downloaded the image and extracted all links with positions relative to the page, size, and URL, and added all links to the page using the link() function, which takes x coordinate, y coordinate, width, height, and URL.
You can add content to the PDF page as per your requirements by exploring the provided functions of PDFKit and how to use them.
After adding content, finally, save the document to a file using the code below:
doc.pipe(require('fs').createWriteStream('file.pdf'));
doc.end();
So, this is how to save the content of a web page element as a PDF file. I hope you find the solution to your problem or learn something by reading this post and find it helpful. Happy Coding!