What are Regex or Regular Expressions in JavaScript and their Usages
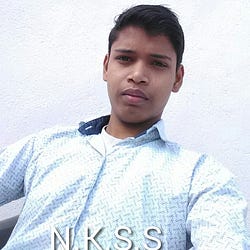
Nitish Kumar Singh
Feb 12, 2024Hello developers! In this blog post, we will explore Regular Expressions (Regex) in JavaScript, exploring their functionalities and applications. We'll learn how to define Regex patterns while adhering to their rules and uncover various ways to utilize them effectively.
I don't have much experience with Regular Expressions because I have not worked with them extensively. However, I do have some knowledge about them, and I'll describe their basic usages at an intermediate level.
Regular Expressions
Regular Expressions, also known shortly as Regex in JavaScript, are strings of characters or patterns written following specific rules. They are used for matching character combinations in strings. We can use the RegExp constructor or a regular expression literal to define a regular expression.
It is essential to follow a rule when writing a regex; otherwise, it is considered invalid and may not work as expected, or it could throw an error. Regular expressions can be used for various string operations, such as searching, matching, replacing, and more. They consist of a combination of characters and metacharacters that define a search pattern.
We can define a regex as shown in the code below:
// Using RegExp object
var regexObject = new RegExp('pattern');
// Using regular expression literal
var regexLiteral = /pattern/;
We can create regular expressions using the RegExp
constructor by passing a pattern string or by using a regular expression literal, which is enclosed in forward slashes (/
), similar to enclosing a string in single or double quotes.
Rule of writing Regular Expressions
When writing regular expressions (regex patterns) in JavaScript, we use a combination of characters and metacharacters to define a search pattern. The following are some common rules and components used in writing regex patterns:
- Literal Characters:
Most characters in a regex pattern match themselves literally. For example, in the regex
/abc/
,abc
will match the string"abc"
in the input and returns true. - Metacharacters:
Metacharacters have special meanings in regex. Examples include:
- . (dot): Matches any character except for a newline.
- ^ (caret): Anchors the regex at the start of the string.
- $ (dollar): Anchors the regex at the end of the string.
- Character Classes:
Square brackets (
[]
) define a character class, allowing us to match any one of the characters inside. For example,[aeiou]
matches any vowel,[0-9]
matches any digit,[a-zA-Z0-9]
matches any alphanumeric. - Quantifiers:
Quantifiers specify the number of occurrences of a character or group. Examples include:
- *: Matches 0 or more occurrences.
- +: Matches 1 or more occurrences.
- ?: Matches 0 or 1 occurrence.
- {n}: Matches exactly n occurrences.
- {n,}: Matches n or more occurrences.
- {n,m}: Matches between n and m occurrences.
- *: Matches 0 or more occurrences.
- Escape Character:
The backslash (
\
) is used as an escape character to treat a metacharacter as a literal character. For example,\.
matches a literal dot("."
). - Groups and Capturing:
Parentheses
()
are used to create groups. They also allow us to capture and reference matched portions of the input. - Alternation:
The pipe
|
allows us to create an OR condition. For example,/cat|dog/
matches either"cat"
or"dog"
. - Wildcard: The dot . matches any single character except for a newline.
- Anchors:
^
and$
are used as anchors to match the start and end of a string, respectively. - Character Escapes:
Backslashes followed by certain characters provide special matching, like
\d
for any digit,\s
for any whitespace character,\w
for any word character, etc. - Modifiers:
Flags such as
i
(case-insensitive),g
(global), andm
(multiline) can be used to modify the behavior of the regex.
We write any type of regex patterns according to our requirements while adhering to the rules mentioned above. For example, to match a decimal number, the correct regex is /\d+.\d+/
, where \d
matches any digit, and to match a dot, we use \.
instead of just dot (.
), as it holds a special meaning in regex.
Usages of Regular Expressions
Regular expressions can be used for various string operations such as searching, matching, manipulation, and more. Some of common usages of regex are listed below.
Searching and Matching: Regular expressions allow us to search for and match patterns within strings. For example:
var str = 'Hello, World!';
var pattern = /Hello/;
console.log(pattern.test(str)); // Output: true
Replacing: We can use regular expressions to replace parts of a string that match a certain pattern:
var str = 'Hello, World!';
var pattern = /Hello/;
var replacement = 'Hi';
var newStr = str.replace(pattern, replacement);
console.log(newStr); // Output: Hi, World!
Validation: Regular expressions are commonly used for validating input, such as email addresses or phone numbers. For example:
var emailPattern = /^[a-zA-Z0-9._-]+@[a-zA-Z0-9.-]+\.[a-zA-Z]{2,4}$/;
var email = 'user@example.com';
console.log(emailPattern.test(email)); // Output: true
Here, the regular expression emailPattern is used to validate an email address.
Splitting: Regular expressions can be used to split strings based on a specified delimiter or pattern:
var str = 'apple,orange,banana';
var fruits = str.split(',');
console.log(fruits); // Output: ['apple', 'orange', 'banana']
These examples only scratch the surface of what regular expressions can do. They provide a flexible and concise way to work with text patterns, making them a valuable tool for tasks like data validation, parsing, and manipulation in JavaScript.
You can read Writing a Regex to Validate an Input String for Setting It as Padding on an Element in JS to explore how to use Regex for validation.
I hope you understand and learn about Regular Expressions in JavaScript and feel this blog post helpful and informative. Happy Coding!