How to Extract Duration of Video Files in Android Using Java
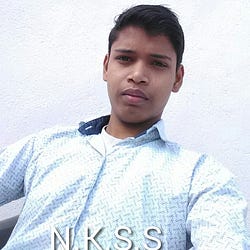
Nitish Kumar Singh
Jan 30, 2024Hello developers! In this blog post, we will explore three methods of extracting the duration of video files in Android development using Java.
Using MediaMetadataRetriever
We can extract the duration of a video file from its path using the MediaMetadataRetriever class of the android.media package. We can also use the Uri of the video file to extract the duration. This method is a little time-consuming, so run the extraction code in a background thread if extracting durations for too many files. The following is the code to extract the duration of a video file.
private int getVideoDuration(String videoPath){
try {
MediaMetadataRetriever retriever = new MediaMetadataRetriever();
retriever.setDataSource(videoPath);
String d = retriever.extractMetadata(MediaMetadataRetriever.METADATA_KEY_DURATION);
int duration = 0;
if (d != null) duration = Integer.parseInt(d);
retriever.close();
return duration;
}catch (Exception e){
// Handle exception
return 0;
}
}
The extracted duration is in milliseconds. This method is good for extracting the duration of only one file. This work can be done in the UI thread.
Using MediaStore
Extracting the duration of video files using MediaStore is very fast, but as we know, it is indexed data of files, so there is a very small chance of the target video file not being currently indexed. The extracted duration is in milliseconds. Use the below code to extract the duration of a video.
public long getVideoDuration(String videoPath) {
try {
Cursor cursor = getContentResolver().query(MediaStore.Video.Media.EXTERNAL_CONTENT_URI,
new String[]{MediaStore.Video.Media.DURATION} , MediaStore.MediaColumns.DATA + " in " + "(?)", new String[]{videoPath}, null);
long duration = 0;
if (cursor != null && cursor.moveToFirst()) {
int durationColumnIndex = cursor.getColumnIndex(MediaStore.Video.Media.DURATION);
duration = cursor.getLong(durationColumnIndex);
cursor.close();
}
return duration;
} catch (Exception e) {
// Handle Exception
return 0;
}
}
If you are using MediaStore for video file information extraction, then video duration can be extracted at the time of file information extraction just by adding the "MediaStore.Video.Media.DURATION" column to the projection array.
Using MediaPlayer
We can use the MediaPlayer class to get the duration of a video. However, creating and preparing a MediaPlayer object can be a bit heavy, so it might not be the most efficient option if we only need to get the duration.
In terms of time, this method of duration extraction takes time between the second and third methods. Below is the code to extract duration using MediaPlayer.
public int getVideoDuration(String videoPath) {
try {
MediaPlayer mediaPlayer = MediaPlayer.create(context, Uri.parse(videoPath));
int duration = mediaPlayer.getDuration(); // in milliseconds
mediaPlayer.release();
return duration;
} catch (Exception e) {
// Handle Exception
return 0;
}
}
All three of these methods can be used to extract the duration of video or audio files, and which method is more efficient depends on the situation in which this task is performed.
I prefer to use MediaMetadataRetriever for extracting the duration of too many files when using the File API. For example, in the development of a Video or Audio player, extract the duration of all video or audio files and store it in SharedPreferences or in a Room Database.
However, if you are doing this task only for one file, then you can use any method. So these are the three ways of video duration extraction. I hope you find the solution to your problem. Happy Coding!