Methods in Java: Why We Use Them, Their Types, and How to Define Them with Examples
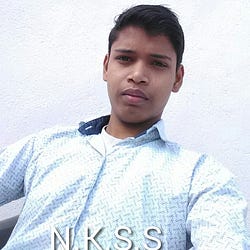
Nitish Kumar Singh
Jan 29, 2024Hello developers! In this blog post, we will explore what Java methods are, why we use them, how to define, declare, or create them, and what their types are.
What are Methods in Java
A method in Java is a way of organizing or structuring code with a name so that we can easily understand the task or action the code performs. While a method can be known as a function, in Java, it is generally referred to as a method.
For example, imagine having hundreds of books without names, just with blank covers. Finding which book is written for what purpose or contains what knowledge becomes a complicated task. However, with names, it becomes easy to identify each book.
This is a simple analogy. In reality, methods in Java solve many problems, including code maintainability, reusability, scalability, and more. Once we create a method, we can use it unlimited times throughout the program or even in other programs by importing it.
In the world of Java, methods are like superheroes, offering a structured approach to building modular, maintainable, and scalable software. The following code is an example of a very simple method that sums two numbers and returns the result.
public int sumTwoNumbers(int a, int b) {
return a + b;
}
Why do we use Java Methods
Java methods are fundamental building blocks that contribute to code organization, reusability, readability, maintainability, and overall software design principles. They enhance the structure of Java programs and are essential for developing modular, scalable, and maintainable software solutions.
As seen in the above books analogy, we use Java methods because they are defined by a specific name that we give as we want. This makes it clear what the method's code does without exploring the actual code.
Java methods are used for various reasons, playing a crucial role in the structure and design of Java programs. Let's explore some key reasons why we use Java methods in the following paragraphs.
Java methods are essential for code organization, breaking down programs into modular units for better readability and maintainability. Each method does a specific task, promoting a structured and systematic codebase. This modular approach also provides code reusability, allowing us to invoke the same functionality across the program or in different projects, reducing code duplication and promoting efficiency.
Methods also contribute to code readability and maintenance by enabling focused updates. With each method addressing a specific functionality, we can easily locate and modify relevant code sections without navigating through the entire program, making the debugging and updating processes simple.
As methods can be defined with parameters, enabling the flexibility to work with different inputs. This parameterization allows methods to perform similar tasks on different data, contributing to the versatility of the code.
Methods allow us to abstract away complex implementations. By encapsulating functionality within a method, the details of how a particular task is accomplished are hidden from the rest of the program, promoting a higher level of abstraction. This is actually a feature of class inheritance, but methods also play an important role.
How to Define Java Methods
Java Methods are defined within a class using the following syntax rules:
[Access Modifier] [Non-Access Modifier] [Return Type] [Method Name] ( [Parameter List] ) [Exception List] {
// Method body
}
The following points describe each component of the method declaration or creation:
- Access Modifier: Specifies the visibility of the method (public, private, protected, or default/package-private). Default means without any access modifier.
- Non-Access Modifier or Modifier: Additional modifiers such as static, final, abstract, synchronized, native, and strictfp. These modifiers are used as required; otherwise, they are optional or defined without them.
- Return Type: Specifies the type of value that the method will return. Use void if the method does not return any value.
- Method Name: The name of the method, which is used to invoke it. Generally, give the name that relates to the task done by the method code.
- Parameter List: A list of comma-separated input parameters (if any) enclosed in parentheses. Parameters are variables used to pass values into the method.
- Exception List: Specifies the exceptions that the method may throw (optional).
- Body of the Method: The actual code enclosed in curly brackets "{}".
The following code shows some examples of methods in Java:
// Simple Method without Parameters:
public void simpleMethod() {
// Method body
}
// Method with Parameters:
public int add(int a, int b) {
return a + b;
}
// Method with Non-Access Modifier:
public static void staticMethod() {
// Method body
}
// Method with Exception Handling:
public void readFile() throws IOException {
// Method body that may throw IOException
}
Types of Methods in Java
Classification of methods in Java is done using various criteria, such as based on non-access modifiers, their characteristics, and functionalities. The following are some common types of methods in Java.
Instance Methods
Defined without the static modifier, can access instance variables (non-static variables) in the method's body code, and Invoked using an object of the class.
public void instanceMethod() {
// Method implementation
}
Static Methods
Defined with the static modifier, cannot access instance variables (non-static variables) in the method's body code, and Invoked using only the class name.
public static void classMethod() {
// Method implementation }
}
Getter and Setter Methods
Accessor (Getter) Methods are used to retrieve the values of private instance variables to provide controlled access to the state of an object. Mutator (Setter) Methods are used to modify the values of private instance variables to maintain encapsulation and control the state of an object.
private String name; // Private instance variable
// Getter method to retrieve the value of 'name'
public String getName() {
return name;
}
// Setter method to modify the value of 'name'
public void setName(String newName) {
this.name = newName;
}
Abstract Methods
Declared in an abstract class or interface without implementation and must be implemented (overridden) by subclasses. This is defined by using abstract modifier and without body.
abstract class Shape {
// Abstract method without a body
public abstract double calculateArea();
}
class Circle extends Shape {
private double radius;
public Circle(double radius) {
this.radius = radius;
}
// Concrete implementation of the abstract method
@Override
public double calculateArea() {
return Math.PI * radius * radius;
}
}
Constructor Methods
Special methods used for object initialization. It is a must-have the same name as the class and no return type. Generally, it is known as Constructor, not a method.
public class User{
private String name;
// Constructor method
public Car(String name) {
this.name= name;
}
}
There are many more methods in Java, each enhancing flexibility. Method Overloading allows multiple methods with the same name but different parameters for versatility. Recursive Methods call themselves, useful for breaking down complex problems. Varargs Methods accept many variable arguments, providing flexibility in parameter passing.
All methods in Java must be defined within a class. This encapsulation promotes code organization, reusability, and follows the object-oriented paradigm. Methods define behavior and are crucial for structuring and modularizing Java programs, contributing to maintainability and scalability.
So, this is Methods in Java, touching on various related questions. I hope you learn and understand all about methods in Java, and the rest of things are automatically understood by practicing and creating different types of methods. Happy Coding!