Pure JS Color Picker - An NPM Color Picker Package
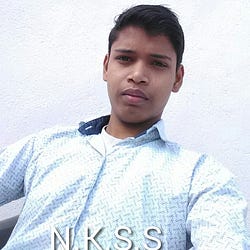
Nitish Kumar Singh
Jan 27, 2024Hello developers! In this blog post, I will describe the npm package "purejs-color-picker", a simple color picker for any type of JavaScript project with RGB and Hexadecimal color models. This package was created by me or other contributors if any.
Try the live demo below:
Features of this color picker:
- Zero dependencies: Built with pure JavaScript without any dependencies.
- Customization: Open the color picker with your own size and position.
- Lightweight: A lightweight color picker under 40KB.
- Simple to Use: To show it, just call the
showColorPicker
function by passing an object with required parameters. - With own CSS: The picker shows in the shadow root with its own CSS in a style tag, so it doesn't conflict with external document CSS.
- Closing Control: You can control whether the picker is dismissed by the user or not.
- More: It shows both RGB color channels and Hexadecimal color codes with an alpha channel. It can be used as an RGB to Hexadecimal converter. Enter RGB values, change it to hex code, and copy it to the clipboard.
Watch the video below for a demonstration:
Install this package via npm:
npm install purejs-color-picker
Import it by a relative path in a pure JavaScript project (if not using any bundler) or from "purejs-color-picker."
import showColorPicker from "...";
// OR
import showColorPicker from "purejs-color-picker";
To show the color picker, call the showColorPicker
function by passing an object with the following properties as required:
- host: An HTMLElement to append the color picker to; the default is the body element of the document. If you pass a host, also pass pickerStyle to override default styles.
- color: The default selected color (RGB or Hexadecimal color string) when showing the color picker.
- handler: A function to listen for changes in the selected color.
- pickerStyle: An HTMLElement to show the color picker based on its position on the document OR a string of inline CSS to show with custom style.
- dismissable: A boolean value "true" or "false" to make the picker auto-dismissable or not auto-dismissable.
The default selected color is red "rgb(255, 0, 0)
" if the color is not passed, and the default pickerStyle
is the following CSS.
.colorPicker {
inset: 0;
max-width: 540px;
min-width: 300px;
position: fixed;
height: fit-content;
z-index: 5000;
background: #fff;
padding: 15px;
box-sizing: border-box;
box-shadow: inset 0 0 3px 2px #5a5a5a;
}
The default value of dismissable
is true
, which means the picker automatically closes when the user clicks outside the picker dialog. When dismissable
is false
, then showColorPicker
returns an object
that has a function as the dismiss
property to close the picker; otherwise, it returns undefined
. The dismiss
function is used only once; after that, it becomes null
.
The following code shows a simple example of showing this color picker on a button click based on the button's position and closes on clicking outside of it.
import showColorPicker from "purejs-color-picker";
const colorPicker = document.getElementById("colorPicker");
colorPicker.addEventListener("click", (event) => {
showColorPicker({
color: event.target.style.background || "rgb(255,0,0)",
handler: (color) => {
event.target.style.background = color;
},
pickerStyle: event.target,
});
});
For source code, contributions, or any issues, go to the GitHub Repository and use it by installing it via NPM.