Creating a Custom Server Using Vercel
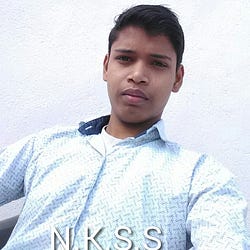
Nitish Kumar Singh
Jan 10, 2024Hello developers! In this blog post, we will create a custom server using Vercel where we can monitor and control each incoming request and send a custom response accordingly.
While Next.js provides full functionality to create a full-stack web app that supports SSR, Streaming, Routing, and API Creation , sometimes we need to create a custom server.
For example, when I integrated Web Stories into my website, I faced many problems with routing in Next.js. I then decided to create a custom server, and by doing so, I solved my routing problem (as I could now control each request). However, this gave rise to more issues, prompting me to use both Next.js and the knowledge of custom server creation to integrate Web Stories.
Let's get started. Initialize the project and install Express.js by running the following commands.
// Initialize project
npm init -y
// Install Express
npm install express
Create an api
folder in the root directory of your project and an index.js
file in the api
folder. Paste the following code into it.
const express = require("express");
const app = express();
app.use((req, res) => {
res.send('Hi from custom server for path '+req.path);
});
// Export the created Express App
module.exports = app;
In the above code, we created an Express app, added a middleware function, and exported it. This is suitable for deployment on Vercel, but if we want to test it locally, we need to add the following code before export and remove the export.
const PORT = 3000;
app.listen(PORT, () => {
console.log(`API listening on PORT ${PORT} `);
});
Create a vercel.json
file in the root directory and paste the following code into it.
{
"version": 2,
"rewrites": [
{
"source": "/(.*)",
"destination": "/api/index.js"
}
],
"trailingSlash": false
}
Here, the source "/(.*)"
means rewrite all incoming requests to the destination "/api/index.js"
.
To deploy your project on Vercel, install the Vercel CLI if not already installed on your computer by running the following command. Create a .gitignore file and add files (.vercel) and folders (node_modules) that you do not want to upload.
npm i -g vercel
Run the vercel
command, and it may ask for login if not logged in, connecting to a Vercel project, or creating a new one. Finally, our project is deployed on Vercel. This deployment is in preview mode, accessible using the given URL. To push it to production, run vercel --prod
.
// deploy in preview mode
vercel
// deploy on production
vercel --prod
Now, for all incoming requests, our attached middleware function is called, having request
and response
objects. In this function, we can prepare data based on the request and send it in the response. Here, we have full control to send custom data headers and cookies.
For static assets, it is recommended by Vercel to create a public folder in the root directory and keep all static assets in it for better performance. Below is what the Vercel Guide says, and you can explore more details at Using Express.js with Vercel.
Serving static files with Vercel allows us to do static asset hoisting and push to our Global Edge Network. We recommend using this approach instead of express.static.
However, our app does not run in a traditional server-like environment because it runs in Serverless Functions.