How to Set up Express JS to Create a Local Server and Serve Static Files along with an HTML File
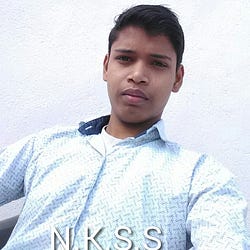
Nitish Kumar Singh
Dec 21, 2023Hello developers! In this blog post, we will create a local server using Express.js and serve some static files from a folder and an index.html file.
Express.js: Express.js is a versatile and lightweight Node.js framework that streamlines web development. It is widely used for its simplicity and modular architecture. Express.js empowers us to build scalable and high-performance applications easily and efficiently.
The following image shows the folder structure of my project that I want to serve using Express.js.
Note: Use Ctrl + = and Ctrl + - to zoom in and out of the folder structure view, and Ctrl + Mouse Wheel to zoom in and out of code in VS Code.
The above folder structure is a project of a sample web story, "Joy of Pets," to learn how to develop web stories using the APM library without any tools or plugins. To learn more and set up Express.js exactly using this project, go to Create your first Web Story on the AMP Docs.
So, let's start setting up Express.js. First, make sure you have Express installed. If not, you can install it using npm:
npm install express
Create a new file for your Express application, like app.js
, and paste the code below in it.
const express = require('express');
const path = require('path');
const app = express();
// Define the directory containing the static files
const publicDirectoryPath = path.join(__dirname, 'assets');
// Serve static files from the 'assets' directory
app.use('/assets', express.static(publicDirectoryPath));
// Serve the 'index.html' file from the root
app.get('/', (req, res) => {
res.sendFile(path.join(__dirname, 'index.html'));
});
// Start the Express server
const port = 3000;
app.listen(port, () => {
console.log(`Server is up and running on port ${port}`);
});
The first three lines of code import the express
and path
libraries to create an Express app object and define paths. In the 4th line of code, we create a path for our assets folder to refer to the assets folder.
After the 4th line of code, we use the use()
, static()
, and get()
functions from Express to serve static files from an assets folder and serve index.html
for the home page ("/"
path).
The use()
function of Express takes a path and a function (middleware) as parameters. It is used to attach and send a response for the specified path using the attached function. In the above code, we pass "/assets"
as the path
and the function returned by the express.static()
function as parameters. In the express.static
function, we pass the path
of the directory from where we want to serve static files.
The get()
function is also used to attach a middleware function that is called for a GET HTTP incoming request for the specified path. The middleware function has access to the request
and response
objects as parameters. So here, we use the sendFile()
function of the response object to send the index.html
file.
Finally, at the bottom of the code, we start the Express server to listen for incoming requests using the listen()
function by passing the port
and a callback function to check if the server started successfully or not. If the server started successfully, then the callback function gets null as a parameter and an error object on any error.
I hope you learn how to set up an Express server to serve some static files and enjoy reading this blog post. Happy coding!