What is URL, Component, Route and Router in Web Development
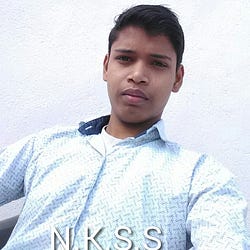
Nitish Kumar Singh
Dec 12, 2023Hello developers! In this blog post, I will discuss what a URL is, its parts and types, components and their types, routes and their types, and routers in web development. Understanding these concepts makes it easier for us to learn single-page application (SPA) libraries and frameworks because they are crucial in SPA development.
URL
A URL (Uniform Resource Locator) is a string of characters that provides a way to identify and locate resources on the internet. It consists of several parts, each serving a specific purpose. For example, https://code.nkslearning.com/blogs/what-is-url-component-route-router-in-web-development?shared_at=facebook#section
is a URL.
A URL consists of the following parts:
- Scheme: The scheme is the first part of the URL and specifies the protocol used to access the resource. For example HTTPS (Hypertext Transfer Protocol), HTTP (Hypertext Transfer Protocol Secure), FTP (File Transfer Protocol) are common schemes.
- Host: The host is the domain name or IP address that identifies the server where the resource is located. For example, in the above example URl,
code.nkslearning.com
is the host. - Port: The port number is an optional part of the URL that specifies a particular port on the server to connect to. If not specified, it defaults to the standard port for the chosen scheme (For example 80 for HTTP, 443 for HTTPS).
- Path: The path identifies the specific resource (file or directory) on the server. In above example URL
/blogs/what-is-url-component-route-router-in-web-development
is path with two segments. - Query String:
The query string is used to pass parameters to the resource. It starts with a "?" and includes key-value pairs separated by
&
. For example, in above URL?shared_at=facebook
is the query string with single key-value pair. - Fragment:
The fragment is an optional part of the URL introduced by a
#
symbol, followed by an anchor name within the HTML document. It is used to link directly to a specific section of a page to directly scroll to that section. For example, in above URL#section
is the fragment.
The following are types of URLs:
- Absolute URL:
Contains the full address of the resource, including the scheme, host, and path. Example:
https://www.example.com/page
. - Relative URL:
Specifies the location of a resource in relation to the current URL. It does not include the scheme or host. Example:
/images/image.jpg
. - Root-Relative URL:
Similar to a relative URL but starts from the root of the domain. Example:
/page/subpage
. - Protocol-Relative URL:
Begins with "
//
" and uses the same protocol (HTTP or HTTPS) as the current page. Example://www.example.com/page
.
Note: In static web application, A URL is exact location of resource; In other words, if a url points to a web page (HTML file) then a file is available at that URL. But in SPA, A URL can be or can not be exact (or real) location of resource, Most of the time it is use as only identifier to a resource and actual resource is stored else where, may be in different or short form.
Component
In general, A component is a small part of a bigger part of a thing. Similarly In web development or SPA development, A component is a small piece or block of code that done a specific task in a big SPA. For example a SPA made by thousands lines of code, so to manage and organize this big code, it is divided into hundreds of small code blocks and each code block done a specific task. These small code blocks called as Component.
We give each component a unique name according to it's task or code, so it can be find easily. A Component may be stored as a different file or in same file of different component. A Component can be small as a single UI or a single logical handler and big as a hole application.
Most of the SPA development libraries and framework built on component-based architecture. This approach offers several advantages, including modularity, reusability, and maintainability. For example React, Vue.js, Angular, Svelte, Ember.js all these are follow component-based architecture and following code show examples of components of some framework.
// React Class Component
import React from 'react';
class MyReactComponent extends React.Component {
constructor(props) {
super(props);
this.state = {
message: 'Hello from React!',
};
}
render() {
return (
<div>
<h1>{this.state.message}</h1>
</div>
);
}
}
export default MyReactComponent;
----------------------------------------------------
// Angular Component
import { Component } from '@angular/core';
@Component({
selector: 'app-my-angular-component',
template: '<h1>{{ message }}</h1>',
})
export class MyAngularComponent {
message: string = 'Hello from Angular!';
}
--------------------------------------------------
<!-- Vue.js Component -->
<template>
<div>
<h1>{{ message }}</h1>
</div>
</template>
<script>
export default {
data() {
return {
message: 'Hello from Vue.js!',
};
},
};
</script>
We can be categorize components in different categories. For example UI components, such as presentational and container components, focus on rendering and managing the visual aspects of the user interface. Non-UI components, like store components, route components and high-order components (HOCs), handle logic, state management, and reusable functionalities.
Route
A Route (Path/URL/Endpoint) is a predefined path or URL endpoint that represents a specific location or endpoint within our SPA application, usually identified by a URL. A Route is associated with a specific component called as Route-Component that should be rendered when the user navigates or visits to that particular URL or Path.
For example a About component is rendered when urer visit at path /about
then /about
path is refer as a route and associated About component refer as Route-Component. But generally the component associated with a route is called as Route like About Route, Blog-Post Route.
Router
A Router in web development is a mechanism or module or component that manages the navigation and routing within a Single Page Application (SPA). It enables the association between specific routes (URL paths) and their corresponding components or views.
The Router monitors changes in the URL and ensures that the appropriate component is rendered based on the current route, allowing for dynamic content updates without reloading the entire page. Routers are a fundamental part of SPAs, facilitating organized navigation and enhancing the user experience by enabling the display of different views for distinct URL paths.
Basically Router monitors changes in URL in it's own way and to manage it's own monitoring behavior, The Router provide us ways to change Routes. For example in Next.js, Router provide two ways of route changing: first we add links through Link component provided Next.js and second is through router object that is available by useRouter Hook.
If we externally change the Route or Path and then Next.js not able to monitor this change. To try this just go to a website that build by Next.js and change the path by running below code in console and see what happens.
history.pushState({},"","/about");
You see that URL is changed but DOM has no effect of this change.
I hope you understand and learn what is URL, Component, Route, Router and more things in web development, and feel this blog post informative and enjoy reading it. Happy Coding!