How to Read and Write Files in Next.js on a Vercel Deployed Website
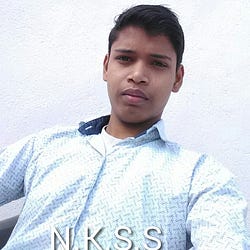
Nitish Kumar Singh
Dec 19, 2023Hello developers! In this blog post, we will explore how to read and write files in a Next.js server component or route handler in the production environment deployed on Vercel.
This is what I learned when I was building a route handler for automatically updating the sitemap of my blog website, such as adding new URLs, updating the lastmod of existing URLs, and deleting a URL. In the development of this route handler in Next.js, I have clearly understood the answers to the following questions.
- Can we read a file in the production environment?: Yes, we can read files in both the development and production environments, but the referencing paths are different in both environments. In my case, the path
./public/sitemap.xml
works fine to refer to the sitemap file under the public folder in development, but it does not work in production. To refer to files in production, we need to use theprocess.cwd()
function to get the current working directory (root directory) and then append the relative path of the file. In my case, to refer to the sitemap file, the path isprocess.cwd() + "/public/sitemap.xml"
. - Can we write a file in the production environment?: No, we can't write a file in the production environment because Vercel uses a serverless function (not a traditional server) to serve files that run on a read-only system. In my case, I successfully built and tested my route handler in development, but when I deployed it, I got
EROFS: read-only file system, open './public/sitemap.xml'
. However, Vercel provides a/tmp
directory to write temporary files in Serverless Functions. You can explore more about it in GitHub Discussion and Vercel Guides.
We can read a file using process.cwd()
to refer to the path and readFile()
from the fs library to read files. The following code is an example of a Route Handler to read a JSON file from the app/data
directory.
// A route handler in app/api/readJSON
import { promises as fs } from 'fs';
export async function GET(req) {
try {
const { searchParams } = new URL(req.url);
let name = searchParams.get("fileName");
const data = await fs.readFile(process.cwd() + '/app/' + name, 'utf8');
return Response.json({ ok: true, results: data });
} catch (error) {
return Response.json({ ok: false, results: { title: "Failed reading JSON", message: error.message } });
}
}
We can create a temporary file in the /tmp
directory in Vercel's Serverless Functions; you can explore a full example in Vercel Guides. Visit Node.js and W3Schools to learn more about the fs (File System) Node.js library.
I hope you learn something by reading this blog post. Happy Coding!