How to Convert a DOM Element to JSON and from JSON to a DOM Element
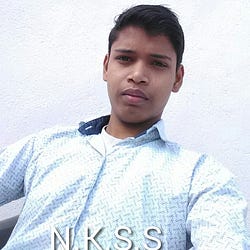
Nitish Kumar Singh
Jan 10, 2024Hello developers! In this blog post, we will create two JavaScript functions to convert a DOM element into a specific JSON format and, conversely, from JSON to a DOM element.
Directly converting a DOM element to JSON using the JSON.stringify()
function is not possible because we encounter the following error when trying this:
Uncaught TypeError: Converting circular structure to JSON
DOM Element to JSON
We will create a JavaScript function to obtain JSON in the following format from a DOM element:
{"nodeType": 1, "nodeName": "DIV",
"attributes": { "class": "container","style": "max-width:1080px;","id": "root"},
"childNodes": [
{"nodeType": 1, "nodeName": "h1",
"attributes": {"style": "font-family:math;"},
"childNodes": [
{"nodeType": 3,"text":"Hello, I'm Title."}
]
}
]
}
The following function returns JSON in the above format.
function getJSONFromDOMElement(element){
if (!element || typeof element !== 'object') {
return null;
}
const json = {};
let a = element.nodeType;
json.nodeType = a;
if(a === 3){
let e = element.textContent;
if(e && e.trim().length > 0) json.text = e;
else return null;
}else if (a === 1) {
json.nodeName = element.nodeName;
let b = element.attributes;
if (b && b.length>0) {
let attributes = {};
for (let i = 0; i < b.length; i++) {
const attribute = b[i];
attributes[attribute.name] = attribute.value;
}
json.attributes = attributes;
}
if (json.nodeName === "svg") {
json.innerHTML = element.innerHTML;
}else{
let c = element.childNodes;
if (c && c.length>0) {
let childNodes = [];
c.forEach((child)=>{
let r = getJSONFromDOMElement(child);
if(r) childNodes.push(r);
});
json.childNodes = childNodes;
}
}
}else return null;
return json;
}
The above function returns a JavaScript object like a parsed JSON, and to get a JSON string, use JSON.stringify()
.
const jsonObject = getJSONFromDOMElement(element);
const jsonString = JSON.stringify(jsonObject);
I use exact names to understand easily, which JSON key is what, like nodeType
, nodeName
, attributes
, and childNodes
—exactly the names in the DOM. But to minimize JSON size, it is good to keep these keys short. For example, t
for nodeType
, n
for nodeName
, and similarly for other keys.
This function returns the exact JSON structure of a DOM element. By using the returned JSON, we can recreate the DOM element back and append it to the DOM.
In this function, I consider only two types of nodes: text and element, but it can be customized according to the use case by adding more if-else
conditions for additional node types.
JSON to DOM Element
The following function creates a DOM element from JSON of the above format.
function createElementFromJSON(json) {
if (!json || typeof json !== 'object') {
return null;
}
if (json.nodeType === 3) {
return document.createTextNode(json.text || '');
} else if (json.nodeType === 1) {
var newNode;
if (json.nodeName === 'svg') {
newNode = document.createElementNS(json.attributes.xmlns || 'http://www.w3.org/2000/svg', json.nodeName);
if (json.attributes) {
for (const attributeName in json.attributes) {
if (json.attributes.hasOwnProperty(attributeName)) {
newNode.setAttribute(attributeName, json.attributes[attributeName]);
}
}
}
newNode.innerHTML = json.innerHTML;
} else {
newNode = document.createElement(json.nodeName);
if (json.attributes) {
for (const attributeName in json.attributes) {
if (json.attributes.hasOwnProperty(attributeName)) {
newNode.setAttribute(attributeName, json.attributes[attributeName]);
}
}
}
if (json.childNodes && json.childNodes.length > 0) {
json.childNodes.forEach((childJSON) => {
const childNode = createElementFromJSON(childJSON);
if (childNode) {
newNode.appendChild(childNode);
}
});
}
}
return newNode;
} else {
return null;
}
}
This function is only used to create a DOM element from JSON if the JSON structure is exactly like the one above.
This blog post's elements are also created using this type of function and stored in the above JSON format. I use these functions for this purpose, but you can modify them according to your requirements.
I hope you learn how to convert a DOM element to JSON and back again to a DOM element and find this blog post informative. Enjoy reading it. Happy Coding!