Converting RGB to Hexadecimal and Hexadecimal to RGB Colors
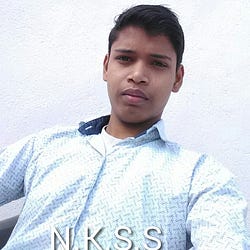
Nitish Kumar Singh
Jan 19, 2024Hello developers! In this blog post we will explore how to convert RGB colors to Hexadecimal (Hex Code) colors and Hex code to RGB colors by creating JavaScript functions.
Before Starting, let's see brief intro of RGB and Hexadecimal Colors. Basically colors made by combining red, green and blue colors with different-different intensity to generate different colors. In RGB colors, intensity represents using digits where 0 represents zero intensity and 255 represents full intensity. In Hexadecimal, zero and full intensity represents by "00" and "FF".
For example white colors generates when these three colors combine with full intensity and represents as "rgb(255, 255, 255)" and "#FFFFFF" in RGB and Hexadecimal colors. To know more about different colors models read my previous blog.
The following function convert RGB color to Hexadecimal color.
function rgbToHex(rgbString) {
const regex = /^rgba?\(\s*([0-9]{1,3})\s*,\s*([0-9]{1,3})\s*,\s*([0-9]{1,3})\s*(?:,\s*(([0-9]*\.)?[0-9]+%?))?\s*\)$/;
const match = rgbString.match(regex);
if (!match) { return null; } // Invalid format
const [, r, g, b, alpha] = match;
const makeInRange = (value, min, max) => Math.min(Math.max(value, min), max);
const toHex = (value) => makeInRange(Math.round(value), 0, 255).toString(16).padStart(2, "0");
let alphaValue;
if (alpha) {
if (alpha.includes("%")) {
// Convert percentage to decimal
alphaValue = parseFloat(alpha) / 100;
} else {
alphaValue = parseFloat(alpha);
}
} else {
// Default alpha value if not provided
alphaValue = 1;
}
const alphaInRange = makeInRange(alphaValue, 0, 1);
// If alpha is 1 then no need to add it in hex code because it is by default 1
const alphaHex = alphaInRange === 1 ? "" : Math.round(alphaInRange * 255).toString(16).padStart(2, "0");
return `#${toHex(r)}${toHex(g)}${toHex(b)}${alphaHex}`;
}
In above code, regex take care of checking rgbString is valid RGB color or not. If rgbString is a valid RGB color then rgbString.match(regex) returns an array containing [rgbString, r, g, b, a] otherwise return null and in next line we also return null. Regex forms in such that it check for both decimal or percentage alpha channel.
In next line, we create two functions makeInRange to make beyond values to in range and toHex to convert each channel of color from RGB to Hex.
After that, we deal with alpha for both form, I mean whether alpha in decimal or in percentage. We not include alpha in hex code if it is equal to 1. And finally returned hex code by combining all colors in hex form.
The following function convert hex color code to RGB color string.
function hexToRGB (hexCode) {
if (/^#([0-9a-fA-F]{3}|[0-9a-fA-F]{4}|[0-9a-fA-F]{6}|[0-9a-fA-F]{8})$/.test(hexCode)) {
const hexWithoutHash = hexCode.slice(1);
const hasShortFormat = hexWithoutHash.length === 3 || hexWithoutHash.length === 4;
const fullHex = hasShortFormat ? hexWithoutHash.split('').map(char => char + char).join(''): hexWithoutHash;
if (fullHex.length === 6 || fullHex.length === 8) {
const a = fullHex.length === 8 ? parseInt(fullHex.slice(6), 16) / 255 : 1;
const alphaHex = a === 1 ? "" : `, ${a}`;
const rgbHex = fullHex.slice(0,6);
const bigint = parseInt(rgbHex, 16);
const r = (bigint >> 16) & 255;
const g = (bigint >> 8) & 255;
const b = bigint & 255;
return `rgb(${r}, ${g}, ${b}${alphaHex})`;
}
}
}
Here also regex take care of validation and after that we remove "#", check for short hex code if it is a short hex code then convert it to full hex code and then make all RGB color channels with alpha in decimal.
Happy coding!