A Comprehensive Guide to Understanding Colors in Web Development
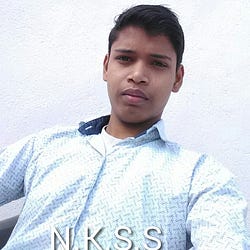
Nitish Kumar Singh
Jan 18, 2024Hello developers! In this blog post, we will explore and understand all aspects of colors in web development. For example, what is color in the context of coding, where and how we use it, and what are the ways to represent colors. Let's dive into these methods of representation.
Colors in Web Development
In web development, we use colors to design or visualize texts, backgrounds, borders, outlines, and other elements to make our website visually appealing and attractive. Colors are applied in CSS, HTML, and JavaScript through various methods, such as RGB colors (rgb(255, 255, 255)
for white) and hex codes (#FFFFFF
for white) in the form of strings in JavaScript and HTML, and using methods in CSS.
We use four models of color representation in web development: RGB (Red, Green, Blue) colors (rgb(255, 255, 255)
, rgb(0, 0, 0)
), hexadecimal color codes (#FFF
, #FF0000
), named colors (red, cyan, yellow), and HSL (Hue, Saturation, Lightness) colors (hsl(120, 100%, 50%)
).
The following code shows the use of colors in HTML, CSS, and JavaScript.
<!-- Setting red background color of div using all four ways in HTML-->
<!-- Named color -->
<div style="background: red;"></div>
<!-- Hexadecimal color -->
<div style="background: #FF0000;"></div>
<!-- RGB color -->
<div style="background: rgb(255, 0, 0);"></div>
<!-- HSL color -->
<div style="background: hsl(0, 100%, 50%);"></div>
/* Setting red background color of div using all four ways in CSS*/
div{
/* Named color */
background: red;
/* Hexadecimal color */
background: #FF0000;
/* RGB color */
background: rgb(255, 0, 0);
/* HSL color */
background: hsl(0, 100%, 50%);
}
// Setting red background color of div using all four ways in JavaScript
const div = document.getElementById("myElement");
// Named color
div.style.background = "red";
// Hexadecimal color
div.style.background = "#FF0000";
// RGB color
div.style.background = "rgb(255, 0, 0)";
// HSL color
div.style.background = "hsl(0, 100%, 50%)";
Named Colors
In web development, named colors stand as the most straightforward and user-friendly method of defining colors. Rather than memorizing hexadecimal values or RGB combinations, we can simply use names that represent specific colors.
For example, we can use red, green, blue, white, and black colors using their names. However, named colors are only available for some standard colors; we cannot use a name for every color.
RGB Colors
RGB colors are a combination of three color channels (components): Red, Green, and Blue, each with a different intensity. Each color component's intensity ranges from 0 to 255.
For example, for red, green, blue, white, and black colors, the combinations are rgb(255, 0, 0)
, rgb(0, 255, 0)
, rgb(0, 0, 255)
, rgb(255, 255, 255)
, and rgb(0, 0, 0)
respectively. We can create every possible color using different intensities of these color channels.
In web development, we can also include an alpha channel (transparency) in RGB to make it RGBA. For example, rgb(255, 0, 0, 50%)
or rgb(255, 0, 0, 0.5)
refers to red color with half transparency.
Hexadecimal Colors
In hexadecimal representation, each color channel (red, green, and blue) is denoted by two hexadecimal digits, ranging from 00
to FF
. For example, the color white is expressed as #FFFFFF
, where FF
represents the maximum intensity for each color channel.
In Hexadecimal color, colors are represented using 6 digits (7 digits including "#") of characters that start with "#" to show it is a hex color code. It is similar to RGB, where the first, second, and third pairs of characters represent Red, Green, and Blue colors. Each pair of hex color code is made using A-F, a-f, and 0-9 characters, where 00 is used to express zero intensity and FF is used to express full intensity.
Some hex color codes have the same character in each pair and can be shortened by removing one character from each pair. For example, the white color hex code (#FFFFFF
) can be used as "#FFF
", where one character is removed from each pair of FF (red), FF (green), and FF (blue). To shorten a hex color code, it must have the same character in each pair, so it is not possible to shorten the every color code.
In hexadecimal colors, we can also include transparency (alpha channel) by including two more digits in the hex color code where FF represents fully opaque, and 00 represents fully transparent. Alpha channel can also be shortened like RGB channels; for example, "#F006
" represents red color with 60% of transparency because 66 is used for 60% transparency.
HSL Colors
The HSL (Hue, Saturation, Lightness) model is indeed used in web development as an alternative color representation alongside RGB and hexadecimal values. HSL provides a different way of specifying colors, offering some advantages in certain situations, especially when dealing with color adjustments and variations.
The HSL colors consist of three values:
- Hue (H): Represents the type of color, ranging from 0 to 360 degrees (red is 0 or 360, green is 120, and blue is 240).
- Saturation (S): Indicates the intensity or purity of the color, expressed as a percentage (0% is grayscale, and 100% is fully saturated).
- Lightness (L): Specifies the brightness of the color, also as a percentage (0% is black, 100% is white, and 50% is normal).
For example, red, green, and blue colors are represented as hsl(0, 100%, 50%)
, hsl(120, 100%, 50%)
, and hsl(240, 100%, 50%)
respectively.
In HSL, we can also include transparency but with a little change; we use hsla()
instead of hsl()
to include the alpha channel. For example, semi-transparent green color is represented as hsla(120, 100%, 50%, 0.5)
.
In CSS, we use names and hex codes for named and hex (hexadecimal) colors, and rgb()
, hsl()
, and hsla()
CSS methods for RGB, HSL, and SHL with alpha colors. In HTML and JavaScript, it's the same as CSS but in the form of strings.
I hope you you understand about different ways of color representations in web development. Happy Coding!