How to Find a Color Between Two Colors in a Linear Gradient
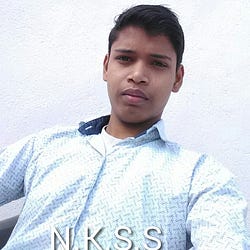
Nitish Kumar Singh
Feb 26, 2025We all, at least once in our life, have interacted with a color picker when using a computer or doing any tech or coding-related work. But have you ever wondered how a color picker works internally and what logic is used to build this tool?
This is what we are going to learn in this post. The most important concept behind a color picker is how to find a color between two colors in a linear gradient. So, let's figure it out step by step.
Understanding Linear Gradients
A linear gradient is a color transition between two or more colors along a straight line, where the colors smoothly change from one to another in a given direction.
Visually, it looks like two given colors at the start and end, with many intermediate colors formed by blending them. In CSS, a gradient from red to blue can be written as:
Linear gradient of Read and Blue
background: linear-gradient(to right, rgb(255, 0, 0), rgb(0, 0, 255));
This means at 0%
, the color is red, and at 100%
, the color is blue. The transition happens smoothly between them, as shown above.
RGB Colors and Their Role
Colors in digital graphics are usually represented by different color models, one of which is the RGB color model. Each color is made up of three components: Red, Green, and Blue, each ranging from 0 to 255. For example:
- Red (255, 0, 0): Red component is max, and the rest are zero.
- Green (0, 255, 0): Green component is max, and the rest are zero.
- Blue (0, 0, 255): Blue component is max, and the rest are zero.
- Purple (128, 0, 128): Red and blue are 128, and green is zero.
We can create many colors by combining different values of these components. Since each component has 256 possible values, we can generate 256 × 256 × 256 = 16,777,216
(16.7 million) different RGB colors.
In a linear gradient, each component (R, G, and B) transitions independently from one color to another. The color picker is based on math that we all studied in 10th and 12th grade.
The Section Formula
Before diving into color calculations, we need to understand the section formula. Given two points A(x₁, y₁) and B(x₂, y₂), a point P dividing AB in the ratio m:n
is given by:
x =
m × x2 + n × x1
m + n
,
y =
m × y2 + n × y1
m + n
This formula helps us find a point between two given points, which is exactly what we need to find colors between two given colors.
Deriving the Parametric Formula from Section Formula
Instead of using m:n
, let's introduce a new parameter t
, which is the fraction of the distance of the target point from the first point (AP) to the total distance (AB). The value of t ranges from 0
to 1
, where 0
means P is at A, and 1
means P is at B. So, t can be written as:
t =
m
m + n
By finding m and n in terms of t and substituting them in the section formula, we get:
x = x1 + t(x2 - x1) , y = y1 + t(y2 - y1)
This is the parametric equation of a line segment, which is key in finding colors between two colors in a linear gradient. So, we can write this formula as: c = c1 + t(c2 - c1).
Finding the Color at a Specific Distance
Now, let’s say we have a linear gradient from Red (255, 0, 0) to Blue (0, 0, 255) over 300px
, and we want to find the color at 145px
from the red stop.
We can find each component of the target color using the formula: c = c1 + t(c2 - c1), where t = 145/300 = 0.4833
.
Now, let’s apply this formula to the RGB channels:
- Red: 255 + 0.4833 x (0 - 255) = 132
- Green: 0 + 0.4833 x (0 - 0) = 0
- Blue: 0 + 0.4833 x (255 - 0) = 123
So, at 145px
, the color is rgb(132, 0, 123).
To calculate the color for a given gradient, with colors c1
, c2
, gradient length l
, and the distance x
, we can use the following function:
function getGradientColor(x, l, c1, c2) {
// Parse RGB values from the color strings
function parseRGB(color) {
return color.match(/\d+/g).map(Number);
}
let rgb1 = parseRGB(c1);
let rgb2 = parseRGB(c2);
// Calculate the parameter t
let t = x / l;
let r = Math.round(rgb1[0] + t * (rgb2[0] - rgb1[0]));
let g = Math.round(rgb1[1] + t * (rgb2[1] - rgb1[1]));
let b = Math.round(rgb1[2] + t * (rgb2[2] - rgb1[2]));
return `rgb(${r}, ${g}, ${b})`;
}
// Example usage:
console.log(getGradientColor(145, 300, "rgb(255, 0, 0)", "rgb(0, 0, 255)"));
// Output: "rgb(132, 0, 123)"
From Simple Math to a Color Picker
We started with a simple question—how to find a color between two colors in a linear gradient—and arrived at the logic behind a color picker.
In a color picker, when we move the color-picking indicator, we track its position on a rectangular color palette where the colors at all vertices are already known. The color at the indicator is found by determining the colors in the x
and y
directions and combining them to get the final result.
I have already written four blogs:
- Building the UI
- Finding the color as the indicator moves
- Positioning the indicator correctly for a given color
- Demonstrating the color picker
You can understand everything behind a color picker by reading these blogs, getting the code from the PureJs-Color-Picker GitHub repository, and implementing it using the PureJs-Color-Picker NPM package.
I hope you found this post informative, helpful, and enjoyable. Happy Coding!