How to Center an HTML Element Vertically and Horizontally in a Parent Container
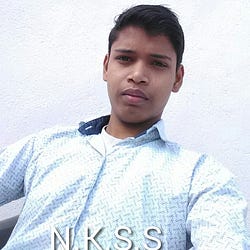
Nitish Kumar Singh
Nov 7, 2023Hello, developers! In this blog post, we will explore various methods for centering an HTML element both vertically and horizontally within its parent container.
Below are the methods to achieve this. We will refer to the parent container as .parent
, and the element that needs to be centered as .child
.
Using Flexbox
You can center an element vertically and horizontally by making the parent element a flexbox container. This approach allows you to center the element without the need to apply any CSS properties directly to the child element. Add the following CSS to the parent container:
.parent {
display: flex;
align-items: center;
justify-content: center;
height: 100vh; /* Adjust width and height as needed */
}
If you only need to center vertically or horizontally, add only one of the following: align-items: center;
for vertical centering or justify-content: center;
for horizontal centering. You can also center elements horizontally with the following CSS:
.child{
display: block;
margin: auto;
}
Using Position and Transform CSS Properties
You can center an element both vertically and horizontally using position and transform CSS properties. Apply the following CSS to the parent container:
.parent {
position: relative;
height: 100vh; /* Adjust width and height as needed */
}
Add the following CSS to child element:
.child {
position: absolute;
top: 50%;
left: 50%;
transform: translate(-50%, -50%);
}
we can also use following CSS instead above CSS for child:
.child{
position: absolute;
margin: auto;
inset: 0;
}
Using CSS Grid
This method utilizes CSS grid to center an element both vertically and horizontally within its parent container. It can also be used to center multiple elements. Apply the following CSS to the parent container without needing to add any CSS to the child element:
.parent {
display: grid;
place-items: center;
height: 100vh; /* Adjust width and height as needed */
}
To center multiple elements, add position: relative;
to the parent and position: absolute;
to all child elements. Below is the CSS to center multiple elements:
.parent {
position: relative;
display: grid;
place-items: center;
height: 100vh; /* Adjust width and height as needed */
}
.child {
position: absolute;
}
In all of the above examples, I have not added width and height properties to the child. You can add these properties to the child elements as needed.
Typically, the first two methods are most commonly used for centering a single element, but the third method is fantastic when you need to center multiple elements. If you only want to center text and not an element, you can use the following CSS to center the text:
.text-element {
/* Adjust width and height as needed */
width: 100%;
height: 50px;
text-align: center; /* Centers text horizontally */
/* Set line-height equal to height for vertical centering */
line-height: 50px;/* Centers text vertically */
}