Exploring RecyclerView in Android App Development: History, Purpose, Classes, and Methods
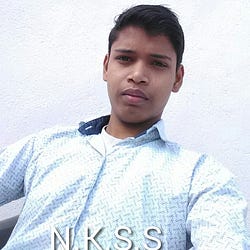
Nitish Kumar Singh
Oct 30, 2023Hello Android Devs, In this blog post, we are going to explore all about RecyclerView in Android App Development. This is an overview of the RecyclerView library.
What is RecyclerView
RecyclerView is an essential component in Android app development that is used to show a large set of data in a more customized and efficient way. It provides more performance, customization, and flexibility than its predecessor, ListView, and GridView. By using it, we can display large data in lists, grids, and staggered layouts and dynamically add, remove, and change these data items. This is used in almost all modern applications where we need to show a large number of data items.
History of RecyclerView
RecyclerView was introduced in 2014 as a part of the Android Lollipop (API 21) release. It was developed to address some of the limitations and performance issues of ListView and GridView. With the migration to AndroidX libraries, RecyclerView is now part of the androidx.recyclerview.widget package.
Why and What for we use RecyclerView
RecyclerView brought several key features, including the ability to create complex layouts with multiple view types, smooth scrolling animations, and an efficient way to recycle views for better performance. It minimizes memory usage and gives us more control over our item's layout design and interaction with views.
By using RecyclerView, we can create vertical and horizontal lists and grids with multiple view types that give us the ability to create section-headered lists created by using lists and grids in combination. We can also create a staggered layout where items are not forced to have the same height or width.
The image below shows a sample of the types of layouts we can create using it.
Now, let's look at which classes and methods are used for various feature implementations. Below are some of the most commonly used classes and their methods in the RecyclerView library.
RecyclerView
This is the main view that performs all the work by itself or with the help of some supporting classes. This view holds all item views, manages scrolling, and takes care of view recycling using the RecyclerView.Adapter
class. To use RecyclerView, we need to create an instance of this class by adding it to our activity_layout.xml file or directly through one of its constructors.
<?xml version="1.0" encoding="utf-8"?>
<FrameLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent">
<androidx.recyclerview.widget.RecyclerView
android:id="@+id/recyclerView"
android:layout_width="match_parent"
android:layout_height="match_parent"/>
</FrameLayout>
// first way to create instance of RecyclerView by adding it to activity_layout.xms file and reference it
RecyclerView recyclerView = findViewById(R.id.recyclerView);
// second way to create instance of RecyclerView by constructor
RecyclerView recyclerView = new RecyclerView(context);
recyclerView.setLayoutParams(new ViewGroup.LayoutParams(ViewGroup.LayoutParams.MATCH_PARENT, ViewGroup.LayoutParams.MATCH_PARENT));
Layout Managers
RecyclerView.LayoutManager
and its built-in subclasses are used to create different types of layout designs like lists, grids, and staggered grids. Below are the subclasses that we use to create different layout designs, and if our needs are not fulfilled by one of these, then we can create our own LayoutManager by creating a subclass of RecyclerView.LayoutManager
.
LinearLayoutManager
: This layout manager is used to create vertical and horizontal lists.GridLayoutManager
: This is used to create vertical and horizontal grids.StaggeredGridLayoutManager
: And this one is used to create vertical and horizontal staggered grids.
Set one of the LayoutManagers as suggested in the code below according to the required list type.
...
// For list
recyclerView.setLayoutManager(new LinearLayoutManager(context));
// For Grid
recyclerView.setLayoutManager(new GridLayoutManager(context,3));
// For staggered grid
recyclerView.setLayoutManager(new StaggeredGridLayoutManager(3,StaggeredGridLayoutManager.VERTICAL));
RecycerView.ViewHolder
A subclass of this class is used to store references to views that are used to display data. Here, we add click listeners to views. Many developers add click listeners in the onBindViewHolder
method of the adapter, but this is not a good practice due to the repetitive calling of this method for data binding by RecyclerView. This class has a method called getAdapterPosition
, which allows us to retrieve the position of the item and the associated data item.
The code below shows an example of a subclass of RecyclerView.ViewHolder:
public static class ItemHolder extends RecyclerView.ViewHolder{
private final TextView title;
public ItemHolder(@NonNull View itemView) {
super(itemView);
title = itemView.findViewById(R.id.title);
}
}
RecyclerView.Adapter
This is the class used by RecyclerView to create item views, bind data while scrolling, determine item sizes, and identify the type of item view for each item. We can think of this class and its methods as the intermediary between RecyclerView and our code. We create a subclass of it or initialize it directly (depending on the use case) and pass this object as a parameter to the setAdapter
method of RecyclerView. This class primarily contains the following methods that we override as needed:
onCreateViewHolder
: This method is called by RecyclerView whenever a new item view is needed. Here, we inflate our designed layout for item views and create an instance of a subclass of RecyclerView.ViewHolder, which we return. This is a required override method.onBindViewHolder
: In this method, we have an object of the holder that contains references to views and the position of the item view. We retrieve the data item based on the position and bind the data to the views. This is a required override method.getItemCount
: Here, we return the number of data items that we are going to display. This is a required override method.getItemViewType
: In this method, we return the type of item views by checking the position or types stored in data items. This method is optional to override and is only overridden when we need to display more than one type of item view.
The code below shows an example of a subclass of RecyclerView.Adapter:
@NonNull
@Override
public ItemHolder onCreateViewHolder(@NonNull ViewGroup parent, int viewType) {
// inflate item view layout and create viewHolder class object and return it
return ...;
}
@Override
public void onBindViewHolder(@NonNull ItemHolder holder, int position) {
// bind data here
}
@Override
public int getItemCount() {
// return size of data
return ...;
}
Above are methods that are used to display data. This class also has more methods like notifyItemInserted
, notifyItemRemoved
, and notifyItemChanged
for dynamically inserting, deleting, and updating data.
Below are some more classes that are used to add additional features to our list or grid.
RecyclerView.ItemDecoration
: This class is used to show dividers between item views. It is also used to add sticky section headers.RecyclerView.ItemAnimator
: This class is used to add animations to item views.ItemTouchHelper
: This class is used to enable drag-and-drop to change the position of item views and swipe to remove items.
The RecyclerView library has many more classes that are used for different features, such as LinearSnapHelper for snapping item views and RecyclerViewPool to improve performance if the same type of layout designs are used in many RecyclerViews. You can learn more about the RecyclerView library by clicking on this link from the official Android Developer Documentation.